mirror of https://github.com/x64dbg/zydis
Various small README tweaks
This commit is contained in:
parent
81b45d39dd
commit
ec174a7efd
57
README.md
57
README.md
|
@ -5,28 +5,15 @@ Fast and lightweight x86/x86-64 disassembler library.
|
||||||
|
|
||||||
## Features
|
## Features
|
||||||
|
|
||||||
- Supports all x86 and x86-64 (AMD64) instructions.
|
- Supports all x86 and x86-64 (AMD64) instructions and [extensions](https://github.com/zyantific/zydis/blob/master/include/Zydis/Generated/EnumISAExt.h)
|
||||||
- Supports pretty much all ISA extensions (list incomplete):
|
|
||||||
- FPU (x87), MMX
|
|
||||||
- SSE, SSE2, SSE3, SSSE3, SSE4.1, SSE4.2, SSE4A, AESNI
|
|
||||||
- AVX, AVX2, AVX512BW, AVX512CD, AVX512DQ, AVX512ER, AVX512F, AVX512PF, AVX512VL
|
|
||||||
- ADX, BMI1, BMI2, FMA, FMA4
|
|
||||||
- Optimized for high performance
|
- Optimized for high performance
|
||||||
- No dynamic memory allocation ("malloc")
|
- No dynamic memory allocation ("malloc")
|
||||||
- Thread-safe by design
|
- Thread-safe by design
|
||||||
- Very small file-size overhead compared to other common disassembler libraries
|
- Very small file-size overhead compared to other common disassembler libraries
|
||||||
- [Complete doxygen documentation](https://www.zyantific.com/doc/zydis/index.html)
|
- [Complete doxygen documentation](https://www.zyantific.com/doc/zydis/index.html)
|
||||||
- No dependencies on platform specific APIs
|
- Absolutely no dependencies — [not even libc](https://github.com/zyantific/zydis/blob/develop/CMakeLists.txt#L32)
|
||||||
- Should compile on any platform with a complete libc and CMake
|
- Should compile on any platform with a working C99 compiler
|
||||||
- Tested on Windows, macOS and Linux
|
- Tested on Windows, macOS, FreeBSD and Linux, both user and kernel mode
|
||||||
|
|
||||||
## Roadmap
|
|
||||||
|
|
||||||
- Language bindings [v2.0 final]
|
|
||||||
- Tests [v2.0 final]
|
|
||||||
- Graphical editor for the instruction-database [v2.0 final]
|
|
||||||
- Implement CMake feature gates. Currently, everything is always included. [v2.0 final]
|
|
||||||
- Encoding support [v2.1]
|
|
||||||
|
|
||||||
## Quick Example
|
## Quick Example
|
||||||
|
|
||||||
|
@ -34,49 +21,52 @@ The following example program uses Zydis to disassemble a given memory buffer an
|
||||||
|
|
||||||
```C
|
```C
|
||||||
#include <stdio.h>
|
#include <stdio.h>
|
||||||
|
#include <inttypes.h>
|
||||||
#include <Zydis/Zydis.h>
|
#include <Zydis/Zydis.h>
|
||||||
|
|
||||||
int main()
|
int main()
|
||||||
{
|
{
|
||||||
uint8_t data[] =
|
uint8_t data[] =
|
||||||
{
|
{
|
||||||
0x51, 0x8D, 0x45, 0xFF, 0x50, 0xFF, 0x75, 0x0C, 0xFF, 0x75,
|
0x51, 0x8D, 0x45, 0xFF, 0x50, 0xFF, 0x75, 0x0C, 0xFF, 0x75,
|
||||||
0x08, 0xFF, 0x15, 0xA0, 0xA5, 0x48, 0x76, 0x85, 0xC0, 0x0F,
|
0x08, 0xFF, 0x15, 0xA0, 0xA5, 0x48, 0x76, 0x85, 0xC0, 0x0F,
|
||||||
0x88, 0xFC, 0xDA, 0x02, 0x00
|
0x88, 0xFC, 0xDA, 0x02, 0x00
|
||||||
};
|
};
|
||||||
|
|
||||||
// Initialize decoder context.
|
// Initialize decoder context.
|
||||||
ZydisDecoder decoder;
|
ZydisDecoder decoder;
|
||||||
ZydisDecoderInit(
|
ZydisDecoderInit(
|
||||||
&decoder,
|
&decoder,
|
||||||
ZYDIS_MACHINE_MODE_LONG_64,
|
ZYDIS_MACHINE_MODE_LONG_64,
|
||||||
ZYDIS_ADDRESS_WIDTH_64);
|
ZYDIS_ADDRESS_WIDTH_64);
|
||||||
|
|
||||||
// Initialize formatter. Only required when you actually plan to
|
// Initialize formatter. Only required when you actually plan to
|
||||||
// do instruction formatting ("disassembling"), like we do here.
|
// do instruction formatting ("disassembling"), like we do here.
|
||||||
ZydisFormatter formatter;
|
ZydisFormatter formatter;
|
||||||
ZydisFormatterInit(&formatter, ZYDIS_FORMATTER_STYLE_INTEL);
|
ZydisFormatterInit(&formatter, ZYDIS_FORMATTER_STYLE_INTEL);
|
||||||
|
|
||||||
// Loop over the instructions in our buffer.
|
// Loop over the instructions in our buffer.
|
||||||
|
// The IP is chosen arbitrary here in order to better visualize
|
||||||
|
// relative addressing.
|
||||||
uint64_t instructionPointer = 0x007FFFFFFF400000;
|
uint64_t instructionPointer = 0x007FFFFFFF400000;
|
||||||
uint8_t* readPointer = data;
|
size_t offset = 0;
|
||||||
size_t length = sizeof(data);
|
size_t length = sizeof(data);
|
||||||
ZydisDecodedInstruction instruction;
|
ZydisDecodedInstruction instruction;
|
||||||
while (ZYDIS_SUCCESS(ZydisDecoderDecodeBuffer(
|
while (ZYDIS_SUCCESS(ZydisDecoderDecodeBuffer(
|
||||||
&decoder, readPointer, length, instructionPointer, &instruction)))
|
&decoder, data + offset, length - offset,
|
||||||
|
instructionPointer, &instruction)))
|
||||||
{
|
{
|
||||||
// Print current instruction pointer.
|
// Print current instruction pointer.
|
||||||
printf("%016" PRIX64 " ", instructionPointer);
|
printf("%016" PRIX64 " ", instructionPointer);
|
||||||
|
|
||||||
// Format & print the binary instruction
|
// Format & print the binary instruction
|
||||||
// structure to human readable format.
|
// structure to human readable format.
|
||||||
char buffer[256];
|
char buffer[256];
|
||||||
ZydisFormatterFormatInstruction(
|
ZydisFormatterFormatInstruction(
|
||||||
&formatter, &instruction, buffer, sizeof(buffer));
|
&formatter, &instruction, buffer, sizeof(buffer));
|
||||||
puts(buffer);
|
puts(buffer);
|
||||||
|
|
||||||
readPointer += instruction.length;
|
offset += instruction.length;
|
||||||
length -= instruction.length;
|
|
||||||
instructionPointer += instruction.length;
|
instructionPointer += instruction.length;
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
|
@ -97,12 +87,13 @@ The above example program generates the following output:
|
||||||
007FFFFFFF400013 js 0x007FFFFFFF42DB15
|
007FFFFFFF400013 js 0x007FFFFFFF42DB15
|
||||||
```
|
```
|
||||||
|
|
||||||
## Compilation
|
## Build
|
||||||
|
|
||||||
|
#### Unix
|
||||||
|
|
||||||
Zydis builds cleanly on most platforms without any external dependencies. You can use CMake to generate project files for your favorite C99 compiler.
|
Zydis builds cleanly on most platforms without any external dependencies. You can use CMake to generate project files for your favorite C99 compiler.
|
||||||
|
|
||||||
```bash
|
```bash
|
||||||
# Linux and OS X
|
|
||||||
git clone 'https://github.com/zyantific/zydis.git'
|
git clone 'https://github.com/zyantific/zydis.git'
|
||||||
cd zydis
|
cd zydis
|
||||||
mkdir build && cd build
|
mkdir build && cd build
|
||||||
|
@ -110,12 +101,16 @@ cmake ..
|
||||||
make
|
make
|
||||||
```
|
```
|
||||||
|
|
||||||
|
#### Windows
|
||||||
|
|
||||||
|
Either use the [Visual Studio 2017 project](https://github.com/zyantific/zydis/tree/master/msvc) or build Zydis using [CMake](https://cmake.org/download/) ([video guide](https://www.youtube.com/watch?v=fywLDK1OAtQ)).
|
||||||
|
|
||||||
## `ZydisInfo` tool
|
## `ZydisInfo` tool
|
||||||
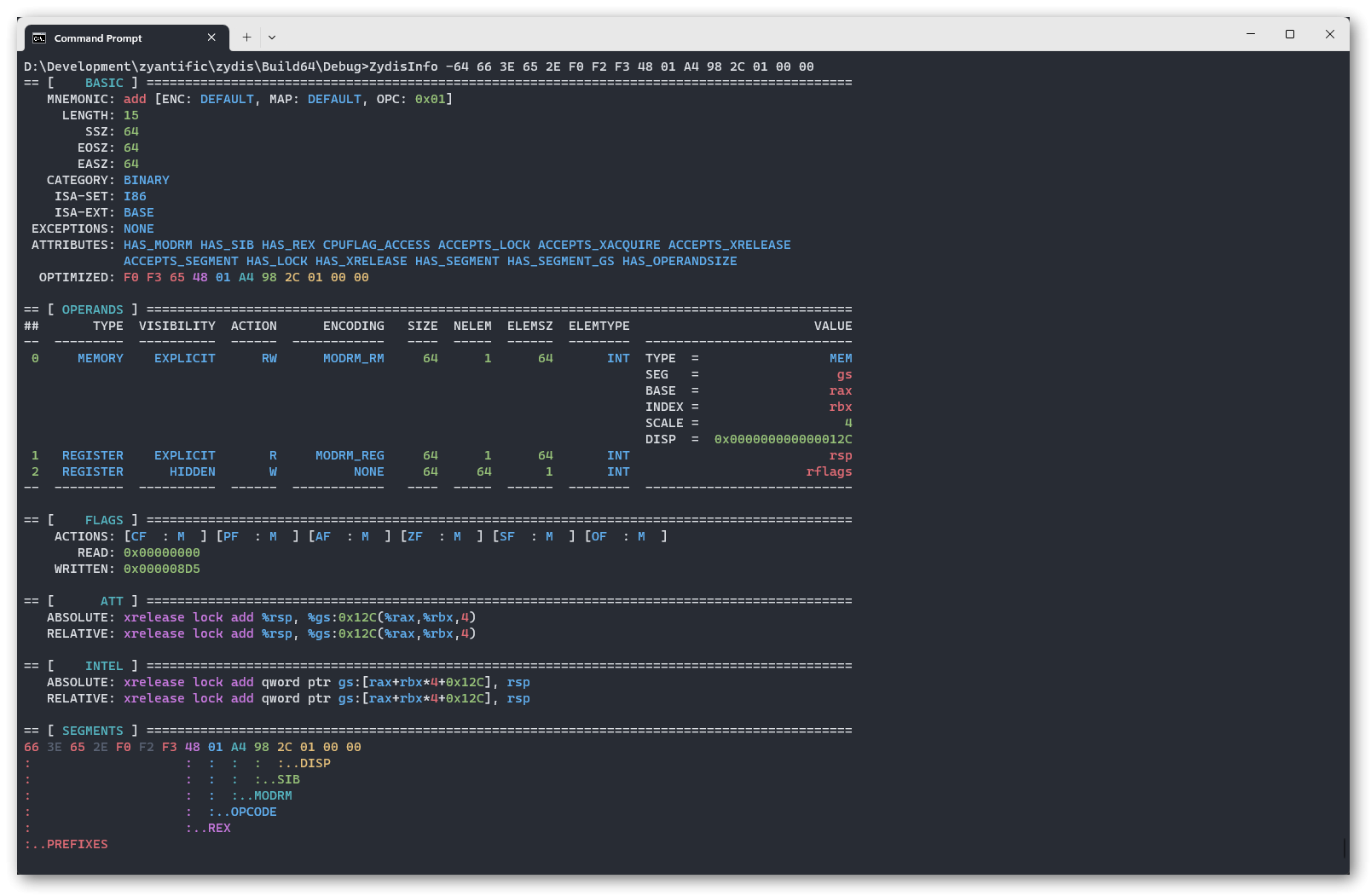
|
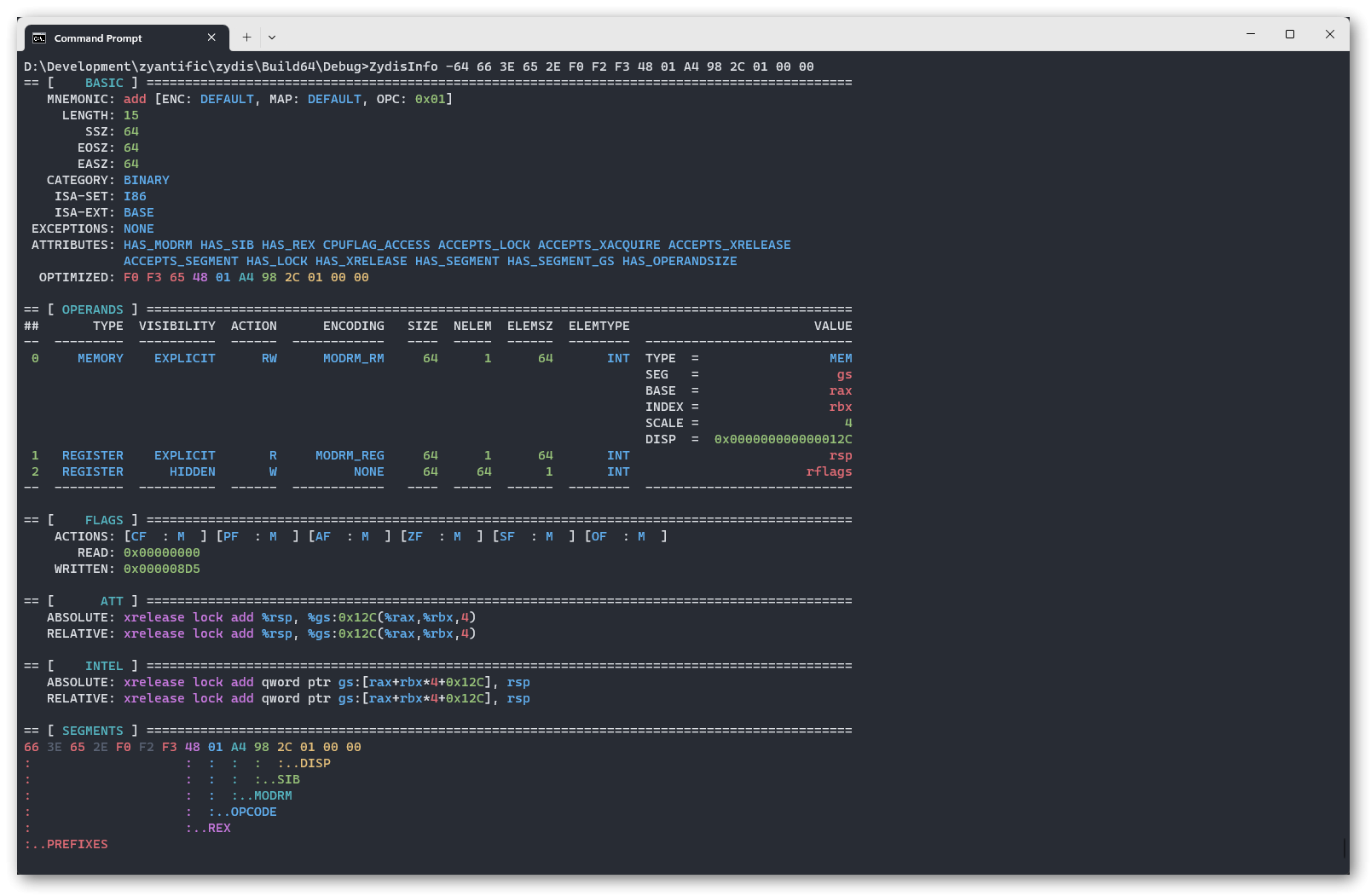
|
||||||
|
|
||||||
## Credits
|
## Credits
|
||||||
- Intel (for open-sourcing XED, allowing for automatic comparision of our tables against theirs, improving both)
|
- Intel (for open-sourcing [XED](https://github.com/intelxed/xed), allowing for automatic comparision of our tables against theirs, improving both)
|
||||||
- LLVM (for providing pretty solid instruction data as well)
|
- [LLVM](https://llvm.org) (for providing pretty solid instruction data as well)
|
||||||
- Christian Ludloff (http://sandpile.org, insanely helpful)
|
- Christian Ludloff (http://sandpile.org, insanely helpful)
|
||||||
- [LekoArts](https://www.lekoarts.de/) (for creating the project logo)
|
- [LekoArts](https://www.lekoarts.de/) (for creating the project logo)
|
||||||
- Our [contributors on GitHub](https://github.com/zyantific/zydis/graphs/contributors)
|
- Our [contributors on GitHub](https://github.com/zyantific/zydis/graphs/contributors)
|
||||||
|
|
Loading…
Reference in New Issue